What’s New With Java 17
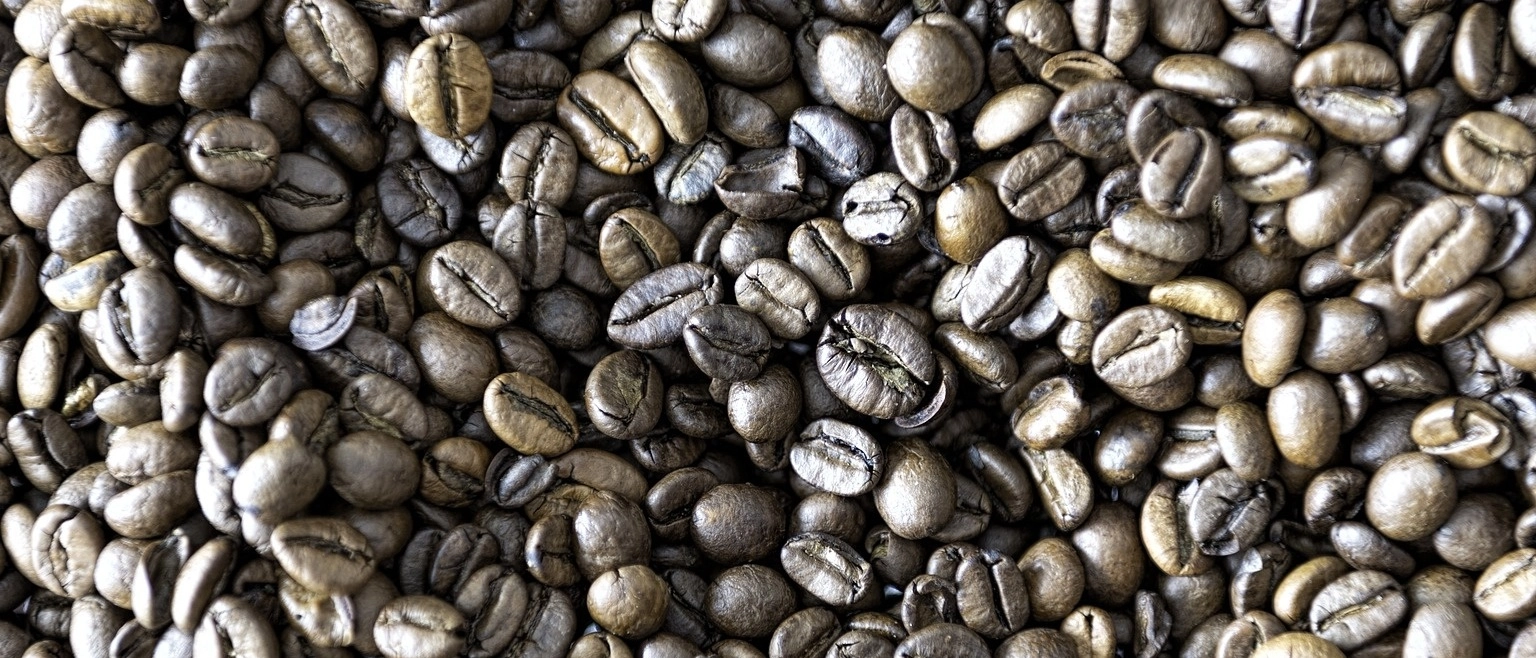
General Information
A new version of Java is here and with it, a whole new set of changes.
One key element of this release is that both Oracle and OpenJDK have determined that this is a long-term support (LTS) release, so it will be a mainstay in the industry for some time. Based on the past releases, there is usually a span of approximately three years between these more significant releases.
With every new version comes new features, changes to existing features, and the deprecation or removal of features that have either become obsolete or simply take too many resources to be worth maintaining compared to the value of the features in question. New Features
As with any new version of Java, there are a plethora of new features and preview features that have become officially implemented. Here’s what’s new and exciting.
Sealed Classes
Arriving as a preview feature previously, this version finalizes sealed classes, making it a standard part of the platform and language with the goal of allowing a class or interface author to control which control bears responsibility for implementation, allow a more definitive way to restrict superclass usage, and enhance pattern-matching by providing improved analysis.
sealed class Protocol permits EmailProtocol, MqProtocol {
record EmailProtocol(Server server, List consumers) implements Protocol { }
record MqProtocol(MessageQueue queue) implements Protocol { }
}
New MacOS Rendering Pipeline
A 2D Java rendering pipeline is now available that uses a new Apple Metal accelerated rendering API for macOS with the intention of providing as good or better performance than OpenGL in certain situations and to provide a failsafe in the event that OpenGL is rendered obsolete.
Enhanced Pseudo-Random Number Generators
This implementation is intended to provide streams of PRNG in order to facilitate easier interchangeable use of interchangeable PRNG algorithms within applications and eliminate duplicate code in existing classes.
New API Features
Fully implemented for Windows and being finalized for other systems, an option is available which provides higher quality images that can be better scaled in high-DPI situations.
A Vector API has been introduced to competently compile at runtime to optimal vector instructions for CPU architectures, providing better performance than scalar computations. API conciseness and clarity is a prominent focus, as is graceful degradation in events where required instructions are not fully supported.
Here is the sample of scalar computation within array elements:
void scalarComputation(float[] a, float[] b, float[] c) {
for (int i = 0; i < a.length; i++) {
c[i] = (a[i] * a[i] + b[i] * b[i]) * -1.0f;
}
}
And equivalent computation using Vector API:
static final VectorSpecies SPECIES = FloatVector.SPECIES_PREFERRED;
void vectorComputation(float[] a, float[] b, float[] c) {
int i = 0;
int upperBound = SPECIES.loopBound(a.length);
for (; i < upperBound; i += SPECIES.length()) {
// FloatVector va, vb, vc;
var va = FloatVector.fromArray(SPECIES, a, i);
var vb = FloatVector.fromArray(SPECIES, b, i);
var vc = va.mul(va)
.add(vb.mul(vb))
.neg();
vc.intoArray(c, i);
}
for (; i < a.length; i++) {
c[i] = (a[i] * a[i] + b[i] * b[i]) * -1.0f;
}
}
Related Packages
A package’s summary page now contains a section describing any “related packages”, which may include parent and sibling packages, as well as any sub-packages, whether or not they are even in the same module.
Configurable Extensions with System Properties
A system property used to disable client TLS extensions and another to disable server TLS extensions have been implemented, and if these extensions are disabled, neither production nor processing will take place in the handshake messages
Content-Specific Deserialization Filters
This addition gives applications the means to configure dynamically selected and context-specific deserialization using a JVM-wide filter factory activated to choose a filter for individual operations.
The intent here is to stabilize what is an inherently dangerous procedure.
Source Details In Error Messages
Enhancements have been made in error reports as it will display the source line for the error, including a line with a caret (^) indicating the specific position on the line. Furthermore, “info” messages now appear on the standard error stream.
MacOS/AArch64 Port
As Apple is planning to transfer Macintosh to AArch65, there will be a functional port of JDK which is intended to improve performance penalties over the inherent macOS Rosetta 2 translator.
Default Strong Encapsulation of JDK Internals
It will no longer be an option to modify the strong encapsulation of internal elements except for select critical internal APIs via a single command-line option, in order to improve the security of the JDK and to encourage developers to use standard APIs.
Always Strict Floating Point Semantics
Now floating-point semantics will always be strict, restoring this mechanic to its previous setting before the introduction of default floating-point modes.
Foreign Function and Memory API
A new API that allows Java programs to interoperate with data and code outside of Java runtime will create an easier to use developmental model, provide enhanced performance, provide a variety of methods to use different kinds of foreign memory, and improve safety by disabled certain operations by default.
MemorySegment segment = MemorySegment.allocateNative(100, newImplicitScope());
MemorySegment is an layer of abstraction that can be described as either off-heap or on-heap memory region. It can be:
- Native segments - allocated via malloc.
- Mapped segments - like mmap allocation.
- Buffer segments - wrapped around memory associated with existing Java arrays or byte buffers, respectively.
DatagramSocket Update
This update provides support for joining multicast groups and the data documentation is updated to explain configurations to join and leave multicast groups.
With these changes, the DatagramSocket API is no longer dependent on the java.net.MulticastSocket API.
Ideal Graph Visualizer Modernization
There are a variety of enhancements which include:
- Block formation, group removal, and node tracking stabilization
- A faster IGV build system, based on Maven
- An updated and improved ranked quick node search
- Improved node categorization and coloring in filter defaults
- IGV Support up to JDK 15
Page Updates for “New API” and “Deprecated”
Now JavaDoc can generate a document to show changes in API and the “Deprecated” page now allows you to view removed features grouped by which version they were removed.
Other New Additions
- An update to java.io.Console to provide a new method for returning the charset for the console
- Context-specific Deserialization Filter implementation
- JDK Flight Recorder Event for Deserialization changes
- System property native.coding as been introduced
- Support for Compiler Blackholes
- Asynchronous Log Flushing in Unified Logging
- Early Access to macOS on ARM systems
- Additional SunPKCS11 configuration properties
Console Charset API
There has been an update to java.io.Console which provides a new method to return the charset for the console.
Derelict or Deprecated Features
Applet API
This API has been deprecated due to a lack or pending lack of support from all browser vendors.
Security Manager
Since this has not been the primary means to protect client-side Java code for a long time, it has been deprecated with no serious plans to design a replacement.
RMI Activation
The Remote Method Invocation Activation mechanism has been obsolete for some time and is, therefore, deprecated while preserving the rest of the RMI.
Experimental AOT and JIT Compiler
The Java-based ahead-of-time and just-in-time compiler are slated for removal based on the significant discrepancy in its usage ratio versus the effort of maintaining the feature. These modules will be eliminated to enhance efficiency.
Other Features Removed Due to Obsoletion
- Socket implementation factory mechanism
- 3DES and RC4 in Kerberos
- JVM TI Heap Functions 1.0
- sun.misc.Unsafe::defineAnonymousClass
- Telia Company’s Sonera Class2 CA Certificate
Should You Get the Upgrade?
You should absolutely get the upgrade if you have not already done so, especially as bug fixes and improvements have been made since its inception and more libraries and applications are being updated with support for Java 17.
As it is a long-term release, you can expect that production environments will be supporting it eventually if they are not already doing so.
Many features have come a long way since the older versions and there are a lot of obsolete technologies that have been removed or upgraded to better and more useful features. The evolution of the platform helps Java remain relevant in a fast-paced world with constant new ways of using and adapting technology.
Even if you prefer an older long-term release, there are plenty of new features in Java 17 that make it worth the while to convert-- and if you’re starting a new project or are just in the initial stages-- it’s a good time to go ahead and make the change before it becomes a complicated endeavor.
Fortunately, there are tools out there such as Docker which can make the transition easier and even perform a lot of the work itself.
Remember that with every Java release, especially long-term releases, there are a plethora of improvements to security and performance that will enhance your experience.
Plus, since these major releases are typically around for about three years, you can expect to get more value out of a transition during a long-term update.